How to update array of objects in Javascript Script ( 4 Ways )
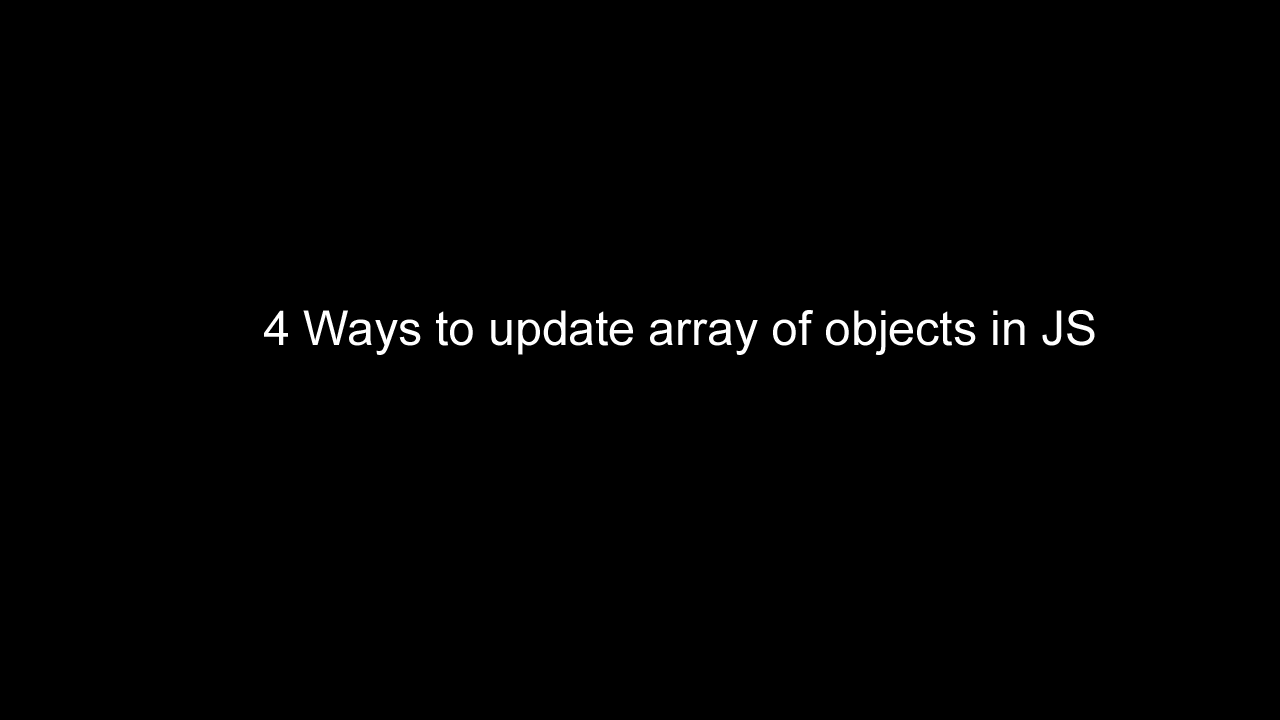
In Javascript, We can update array of objects using 4 ways
let myArray = [
{id: 0, name: "Test"},
{id: 1, name: "Propert"},
{id: 2, name: "Dictonary"},
{id: 3, name: "Book"}
]
Here is the sample array
1. Update by using index, if you know already:
//update by index myArray[0].name ="Updated"; console.log(myArray)
[{
id: 0,
name: "Updated"
}, {
id: 1,
name: "Propert"
}, {
id: 2,
name: "Dictonary"
}, {
id: 3,
name: "Book"
}]
2. Update based on condition using map function
//update based on condition
const arr = myArray.map(e=> {
if(e.id ==2)
{
e.name = "DictonaryUpdated"
return e
}
else return e
})
console.log(arr)
[{
id: 0,
name: "Updated"
}, {
id: 1,
name: "Propert"
}, {
id: 2,
name: "DictonaryUpdated"
}, {
id: 3,
name: "Book"
}]
3. Using Loadash Method :
var object = { 'a': [{ 'b': { 'c': 3 } }] };
_.update(object, 'a[0].b.c', function(n) { return n * n; });
console.log(object.a[0].b.c);
// => 9
4: Using Splice method
var arr2 = myArray.splice(2, 0, {id:6, name: "spp"}, {id:7, name: "spp2"});
console.log(myArray)
[{ id: 0, name: "Test" }, { id: 1, name: "Propert" }, { id: 6, name: "spp" }, { id: 7, name: "spp2" }, { id: 2, name: "Dictonary" }, { id: 3, name: "Book" }]